Description
A Visual Studio extension that parses a SQL Server CREATE TABLE definition file and generates SQL Server Stored Procedures, ASP .Net Model, Service, and Controller code, and Angular Model, Service, and Components code... all with a few clicks.
Available on the Visual Studio Marketplace
Operation
- Right-click on a *.sql file that contains a CREATE TABLE statement, similar to the following:
CREATE TABLE [dbo].[SampleObject]
(
[Id] INT NOT NULL PRIMARY KEY,
[Name] VARCHAR(50) NOT NULL,
[Comments] VARCHAR(max) NULL,
[Price] DECIMAL(20,2),
[IsEnabled] BIT DEFAULT((1)) NOT NULL,
[TypeId] INT NULL,
[StartTimestamp] DATETIME NULL
)
- Select the "Full Stack Code Gen" command:
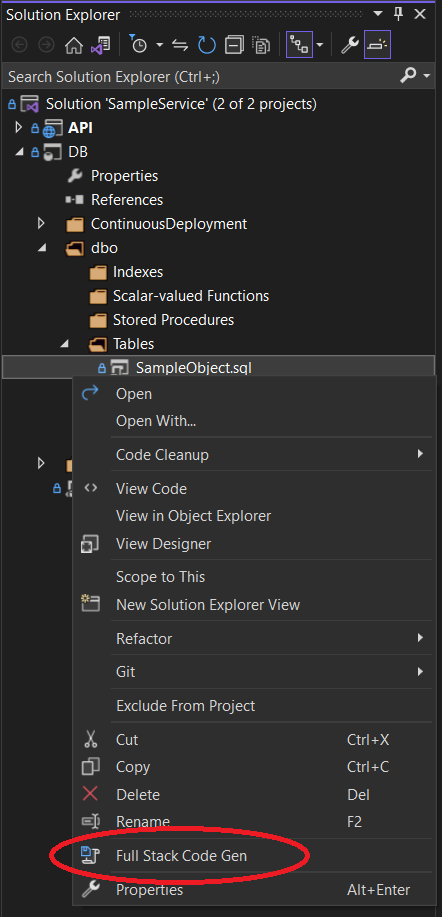
- Select the appropriate options on the "Full Stack Code Gen" tool window and click "Preview Code":
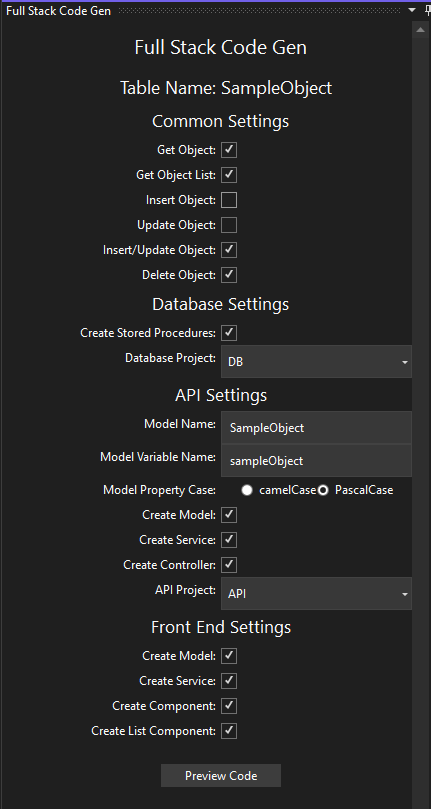
- Smile :)
Common Settings
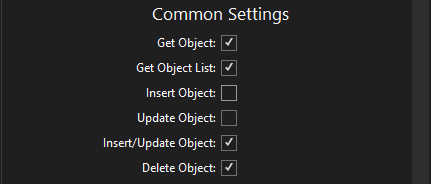
The Common Settings selections dictate which stored procedures and methods are created for the other settings selections.
For example: if "Get Object" is selected in Common Settings, the following is the code that will be generated if "Create Stored Procedures (Database Settings)", "Create Model (API Settings)", "Create Service (API Settings)", and "Create Controller (API Settings)" are selected:
////////////////////////////// SampleObject - Get Stored Proc //////////////////////////////
CREATE PROCEDURE [dbo].[uspGetSampleObject]
(
@Id INT
)
AS
BEGIN
SET NOCOUNT ON;
SELECT
[Id],
[Name],
[Comments],
[Price],
[IsEnabled],
[TypeId],
[StartTimestamp]
FROM [dbo].[SampleObject]
WHERE
[Id] = @Id;
END;
GO
////////////////////////////// SampleObject - Model //////////////////////////////
using FluentValidation;
using System;
using System.Collections.Generic;
namespace API.Model
{
public class SampleObject
{
public int Id { get; set; }
public string Name { get; set; }
public string Comments { get; set; }
public decimal Price { get; set; }
public bool IsEnabled { get; set; }
public int? TypeId { get; set; }
public DateTime? StartTimestamp { get; set; }
}
public class SampleObjectValidator : AbstractValidator<SampleObject>
{
public SampleObjectValidator()
{
RuleFor(p => p.Id)
.NotEmpty()
.WithName("Id")
.WithMessage("{PropertyName} is required.");
RuleFor(p => p.Name)
.NotEmpty()
.WithName("Name")
.WithMessage("{PropertyName} is required.")
.Length(0, 50)
.WithMessage("{PropertyName} cannot be longer than {MaxLength} characters.");
}
}
}
////////////////////////////// SampleObject - Service //////////////////////////////
using API.Model;
using Dapper;
using Microsoft.Extensions.Options;
using System.Collections.Generic;
using System.Data;
using Microsoft.Data.SqlClient;
using System.Linq;
using System.Threading.Tasks;
//Startup:
//services.AddScoped<SampleObjectService>();
namespace API.Services
{
public class SampleObjectService
{
private AppSettings appSettings;
public SampleObjectService(AppSettings appSettings)
{
this.appSettings = appSettings;
}
public async Task<SampleObject> GetSampleObjectAsync(int sampleObjectId)
{
using (SqlConnection cn = new SqlConnection(appSettings.DBConnection))
{
var query = await cn.QueryAsync<SampleObject>($"dbo.uspGetSampleObject"
, new
{
@Id = sampleObjectId
}
, commandType: CommandType.StoredProcedure);
return query.FirstOrDefault();
}
}
}
}
Common Settings Descriptions:
- Get Object: Generates code to retrieve a single object.
- Get Object List: Generates code to retrieve a list of objects.
- Insert Object: Generates code to create an object.
- Update Object: Generates code to update an object.
- Insert/Update Object: Generates code to save an object (the stored procedure will determine if the object exists and either inserts or updates the object.)
- Delete Object: Generates code to delete the object.
Database Settings
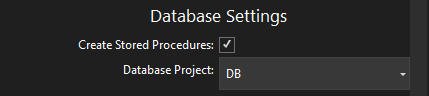
Quick Note: If you are developing against a SQL Server Database, I would thoroughly suggest using a SQL Server Database project. And, if you're going to use a SQL Server Database project, you might as well use the best: SSDT Continuous Deployment-Enabled project template (Kudos to Radoslav Gatev! Wonderful job!)
Database Settings Descriptions
- Create Stored Procedures: Creates stored procedures for all enabled selections in Common Settings.
API Settings
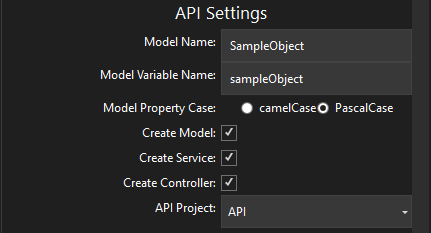
Another few Quick Notes:
- The code generated for the API uses the following libraries:
-
I've also hosted a VS template for the API project at: Full Stack API Template It has a couple of other goodies like:
- Swagger UI
- Serilog.AspNetCore
- Strongly Type Configuration Objects (Kudos to Andrew Lock! Great stuff!)
API Settings Descriptions
- Model Name: Name used for the Model class of the object.
- Model Variable Name: Name used for the variables in the Service and Controller classes.
-
Model Property Case:
- camelCase: All properties of the Model will be formatted in camelCase (meaning, all properties will start with a lowercase letter)
- PascalCase: All properties of the Model will be formatted in PascalCase (meaning, all properties will start with an uppercase letter)
- Create Model: Generates code for the Model class, using the selections above.
-
Create Service: Generates code for a Data Service to interact with the database.
- Please Note: You will have to register the service by adding the commented snippet above the namespace in the service to the ConfigureServices section of the Startup.cs or Program.cs:
//Startup: //services.AddScoped<SampleObjectService>();
- Create Controller: Generates code for an API Controller that uses the Data Service.
- API Project: This selection is used to determine the name of the project to add to the namespaces for the generated code. If no project is selected, "API" is used as the default project name.
Front End Settings
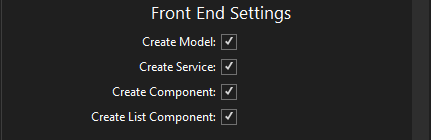
Front End Settings Descriptions
- Create Model: Generates code for a Typescript Model class, based on the database object.
- Create Service: Generates code for a Typescript Service class that maps to the API Controller actions.
- Create Component: Generates Typescript code for an Angular Component and HTML elements for all properties of the Model.
- Create List Component: Generates Typescript code for an Angular Component and HTML Table elements for all properties of the Model.
Configuration
Universal Settings
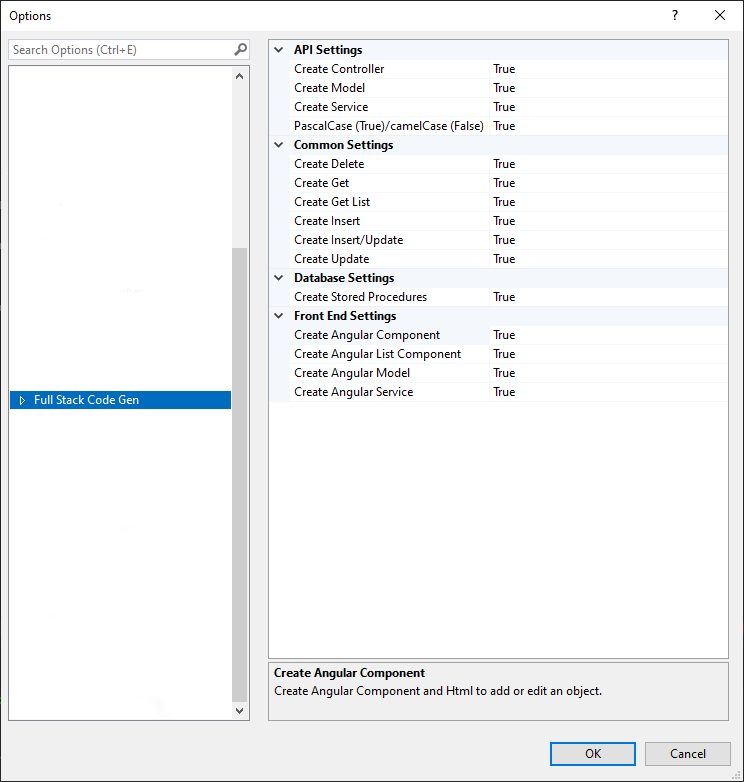
The Universal Settings can be found under Tools -> Options..., Full Stack Code Gen. The Universal Settings will used as the default settings if the Solution Settings config file cannot be found.
Solution Settings
After clicking "Preview Code", the current selections and settings are saved locally to a JSON file (FullStackCodeGen.config) at the root of the Solution project.
FullStackCodeGen.config:
{
"IsCreateGet": true,
"IsCreateGetList": false,
"IsCreateInsert": true,
"IsCreateUpdate": true,
"IsCreateInsertUpdate": false,
"IsCreateDelete": false,
"IsCreateStoredProcedures": true,
"DbProjectName": "DB",
"IsCreateModel": true,
"IsPascalCase": true,
"IsCreateService": true,
"IsCreateController": true,
"ApiProjectName": "API",
"ModelName": "SampleObject",
"ModelVariableName": "sampleObject",
"IsCreateAngularModel": true,
"IsCreateAngularService": true,
"IsCreateAngularComponent": false,
"IsCreateAngularListComponent": false,
"FrontEndProjectPath": ""
}